Get to know our API. If you are looking for our no-code application, visit https://app.customgpt.ai/
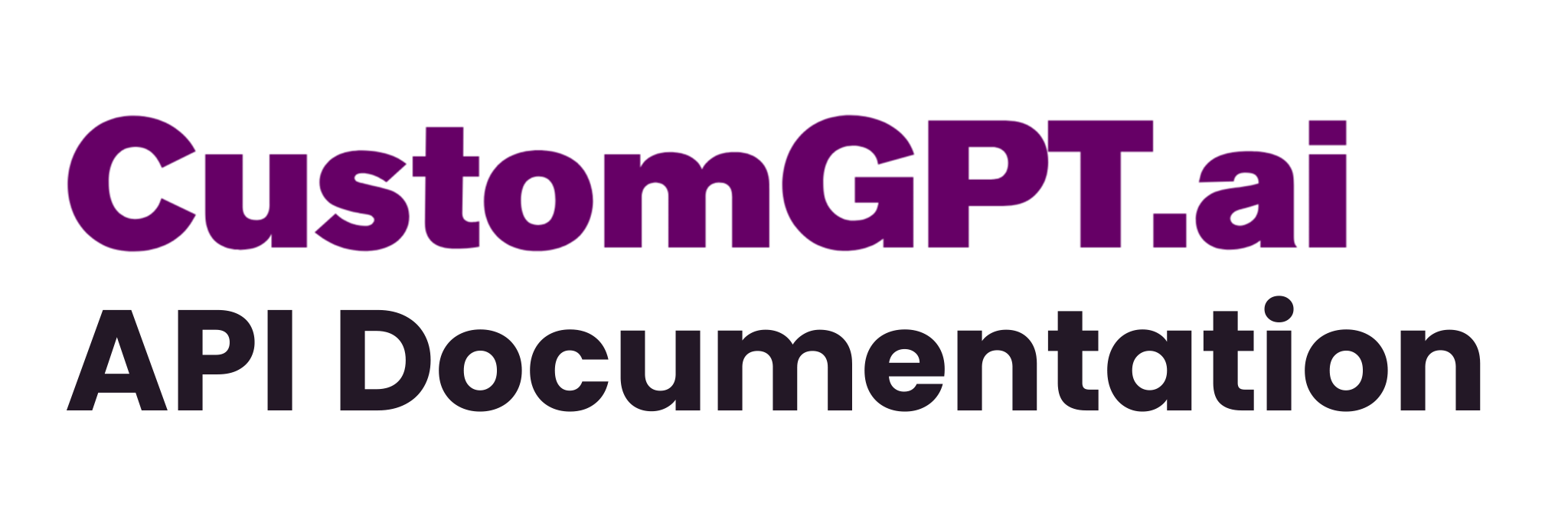
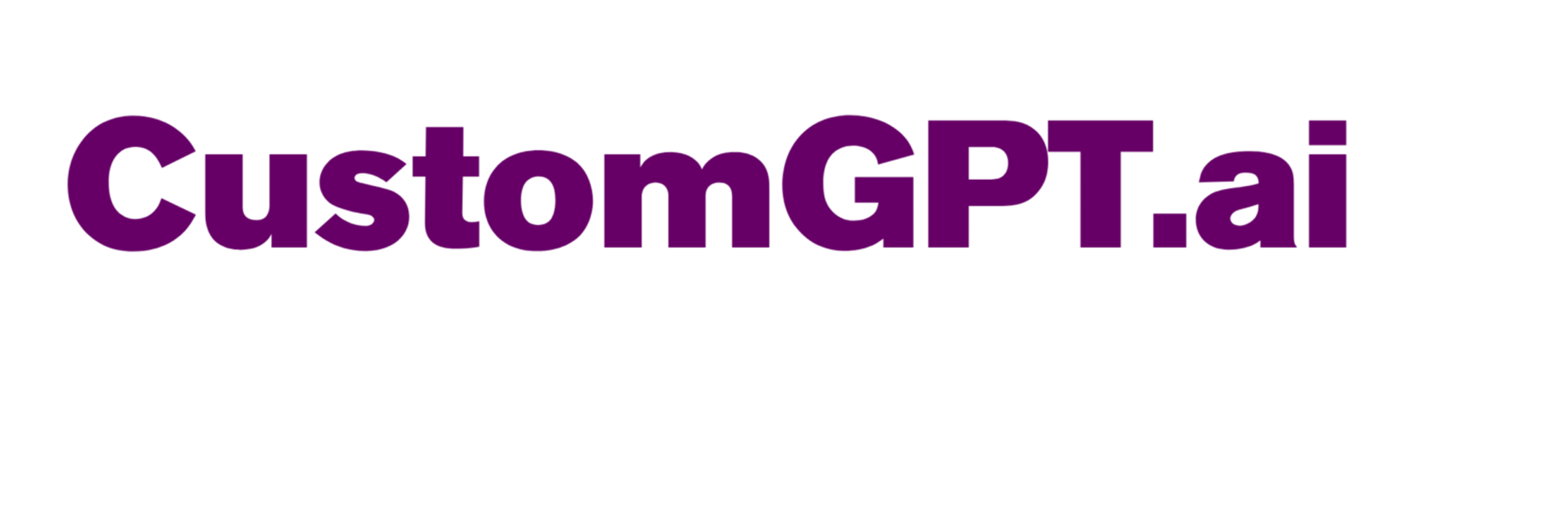
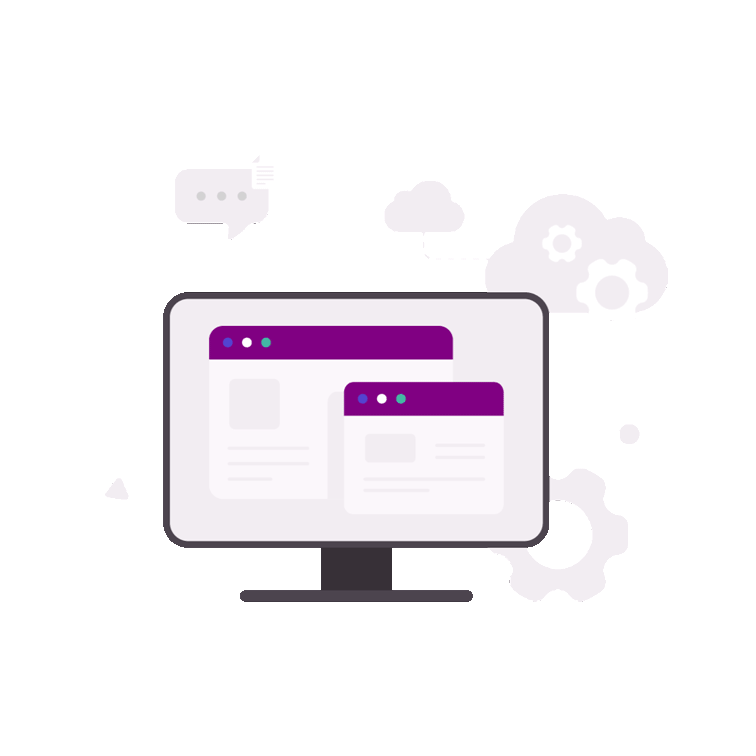
Jump In
You can interact with our API via HTTP requests using any language, or via our dedicated Python SDK. To see some more examples, feel free to look at our cookbooks.
To Install our Python SDK, run this command:
pip install customgpt-client
API Terminology: Agents vs. Projects
In the CustomGPT.ai API, agents are referred to as projects in endpoint URLs, request/response structures, and parameters. While our platform has fully transitioned to using the term agents across the UI and documentation, the API retains the legacy terminology for backward compatibility.
When interacting with the API, any reference to "projects" in endpoints, request bodies, query parameters, or responses corresponds to "agents" in the current system.
API Keys
To start using our Python SDK or make HTTP requests, you'll need a CustomGPT API key for authentication. Read more about our API keys here, otherwise, you can find or create your key from the API tab in My Profile, accessible by clicking the circle in the top right of the application page. Once you have your key, all HTTP requests should include the key in an authorization HTTP header, as shown below:
'authorization: Bearer Your_CustomGPT_API_KEY'
Remember: Your API Key is private. You should not share it with anyone! This includes making sure you are not exposing it through code. You should route your traffic through your own backend where you can protect your key.
Requests
Get familiar with how to make an API call. Below, you will find two of the most common methods for making your API calls, each with their own benefits.
- curl: Curl is easy to set up, as it comes with most operating systems by default. It is a popular command line tool among developers for making HTTP requests due to the minimal setup required, but it is limited in its functionality compared to other programming languages.
- Python: Python is an extremely popular programming language due to its wide array of applications in development and ease of use. CustomGPT offers a Python SDK for further simplification, but you can also make HTTP requests with it directly, as shown below.
curl --request POST \
--url https://app.customgpt.ai/api/v1/projects \
--header 'accept: application/json' \
--header 'authorization: Bearer API Key' \
--header 'content-type: multipart/form-data' \
--form sitemap_path=sitemap \
--form project_name=projectID
import requests
url = "https://app.customgpt.ai/api/v1/projects"
payload = "-----011000010111000001101001\r\nContent-Disposition: form-data; name=\"project_name\"\r\n\r\nprojectID\r\n-----011000010111000001101001\r\nContent-Disposition: form-data; name=\"sitemap_path\"\r\n\r\nsitemap.path\r\n-----011000010111000001101001--"
headers = {
"accept": "application/json",
"content-type": "multipart/form-data; boundary=---011000010111000001101001",
"authorization": "Bearer API Key"
}
response = requests.post(url, data=payload, headers=headers)
print(response.text)